Lab 8 - Stunts
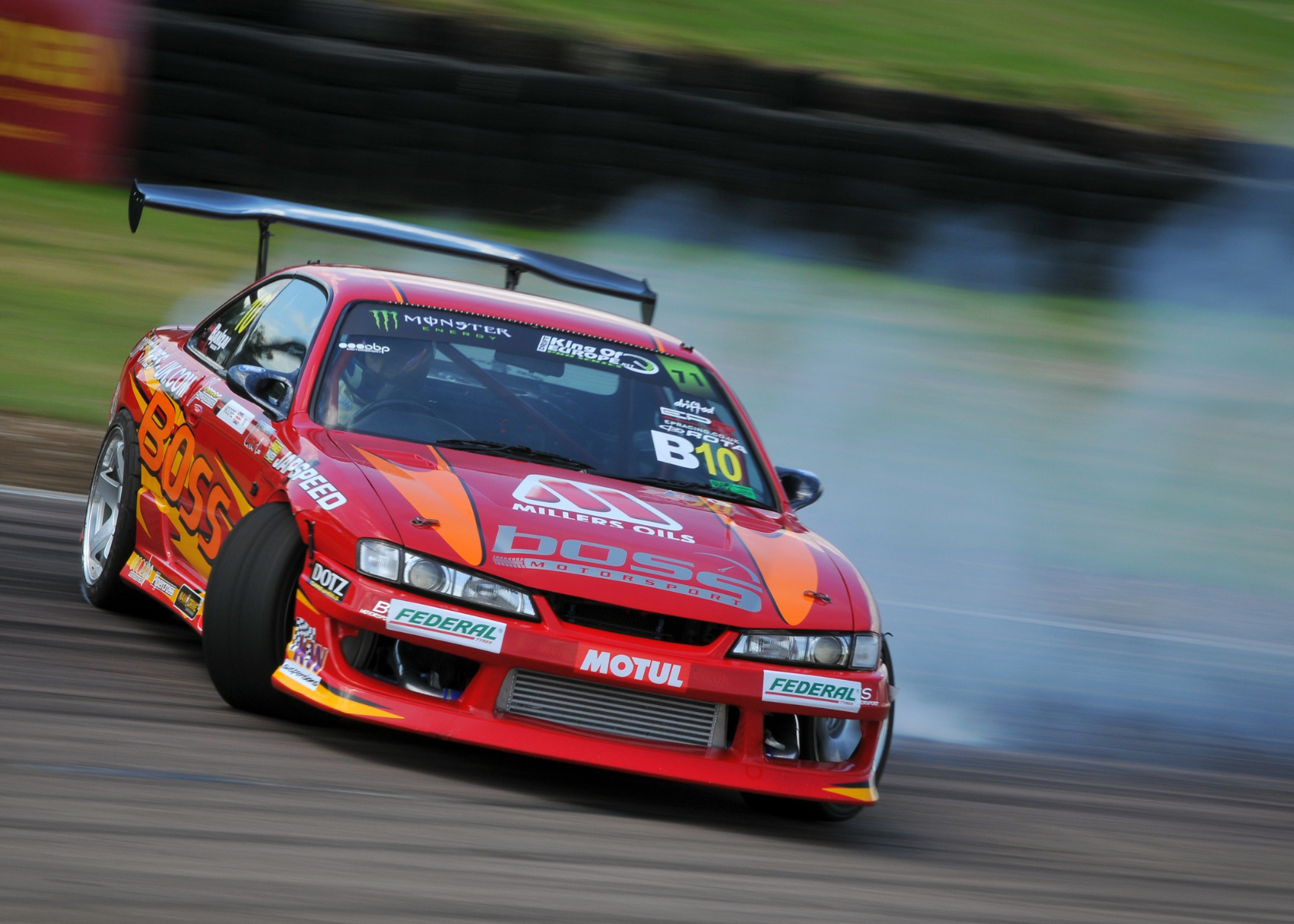
The objective of Lab 8 is to perform a Stunt with the robot.
Prelab
There is no prelab for this lab.
Tasks
The goal of this lab is to perform one of two stunts:
- A. Position Control
- B. Orientation Control
I chose to do Task B: Orientation Control. This tasks consists of driving the car towards the wall, and when it is 1m away from it, do a 180 dregree turn, as fast as posible.
1. Driving towards the wall
Initially, the robot drives in straight line towards the wall at a base_speed
of 50% (remember from Lab 4, that the speed was normalized from 1% to 100% in the working range of the PWM for the motors). When the robot gets close to the wall (distance set with Bluetooth in distanceLimit
), it will initiate the Orientation Controller. The distance towards the wall is obtained with extrapolation; this was proven to be more efective in Lab 5.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
//Obtain distance data or estimate
if(distanceSensorA.checkForDataReady()){
//Read Distance from TOF
}else{
//Extrapolate distance
}
if(stunt.curr_distance < stunt.distanceLimit){
stunt.run_drift = true;
}
2. Orientation Control
Once the robot gets close to the wall, the orientation controller will turn on, turning the robot 180 degrees. This controller is the same as it was implemented in Lab 6, with a few modifications:
- If the error of the controller is 0 for more than 5 loop iterations, we can consider that the robot has successfully turned 180 degrees, so we can turn off the controller.
1
2
3
4
5
if(stunt.last_n_error_0 >= 5){
stunt.pid = 0;
stunt.run_test = false;
stunt.run_drift = false;
}
- Whenever the controller is turned off, the robot will run for half a second more in a straight line, at
base_speed
, and then turn off completely.
1
2
3
4
5
6
7
8
if(stunt.run_test){
setSpeed(stunt.base_speed + stunt.pid, stunt.base_speed - stunt.pid);
}else{
setSpeed(stunt.base_speed + stunt.pid, stunt.base_speed - stunt.pid);
delay(600);
stunt.base_speed = 0;
brake();
}
As it can be seen from the code above, the speed set to the robot is always the base_speed
, plus the input from the Orientation Controller.
3. Stunts!
With the code ready, the robot was set to perform these drifts. As a safety measure, some protection was placed in front of the wall, in case the robot crashes. A lot of testing was made, with too many crashes in different locations. However, the robot was strong enough and didn’t suffer any damages.
Most bloopers and failed runs are included in this YouTube playlist.
After many trials, the correct Kp, Ki and Kd values were found. With Kp = 0.55, Ki = 0.3, and Kd = 0.5, the robot was able to perform the stunts. We can see the results in the following videos, with the data from the robot below them.